A Comprehensive Guide to Full-Stack Development with React and Python
Rohan Roy
Apr 7, 2025
Web Development






Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
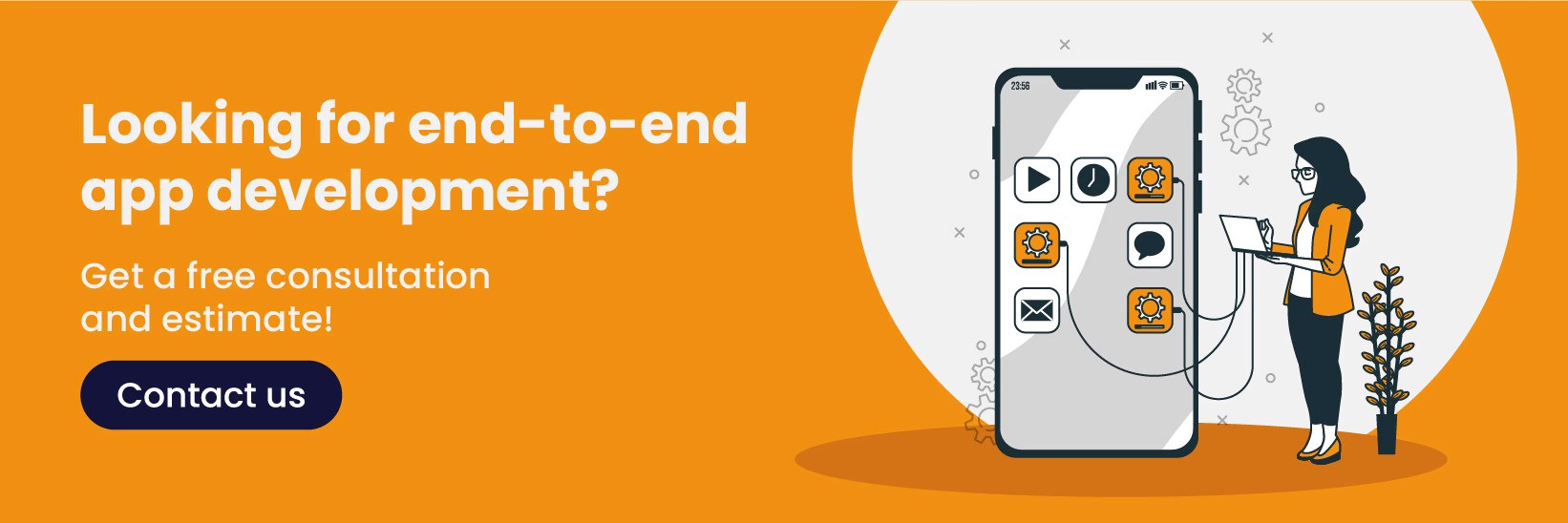
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
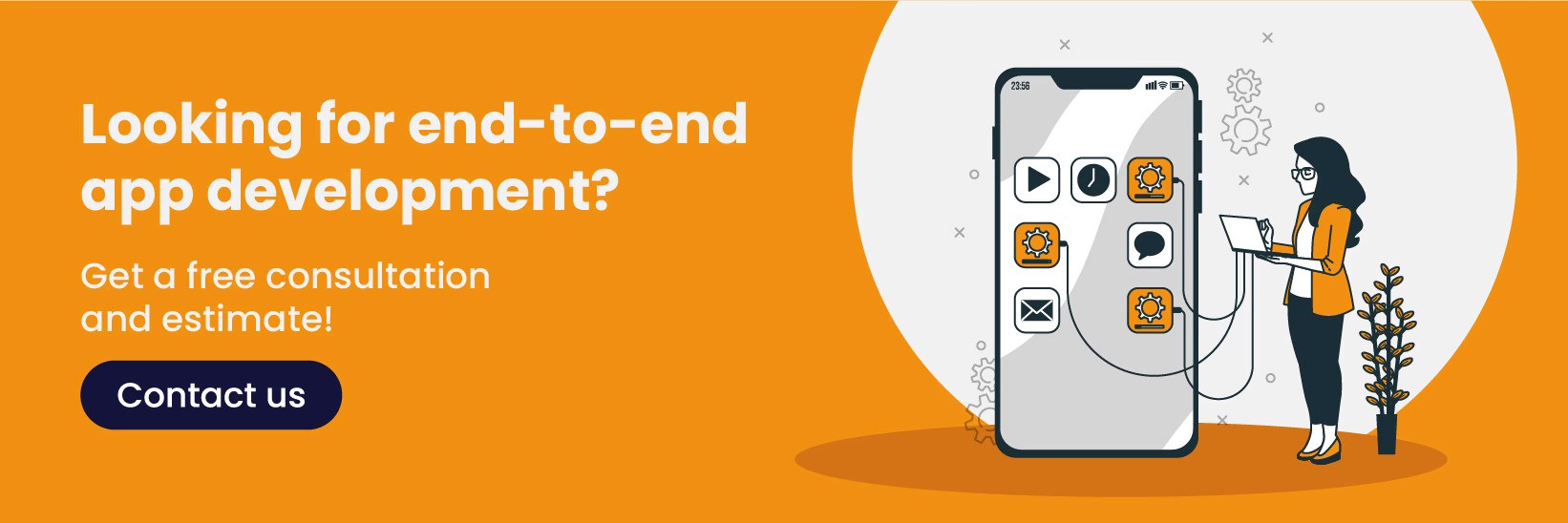
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
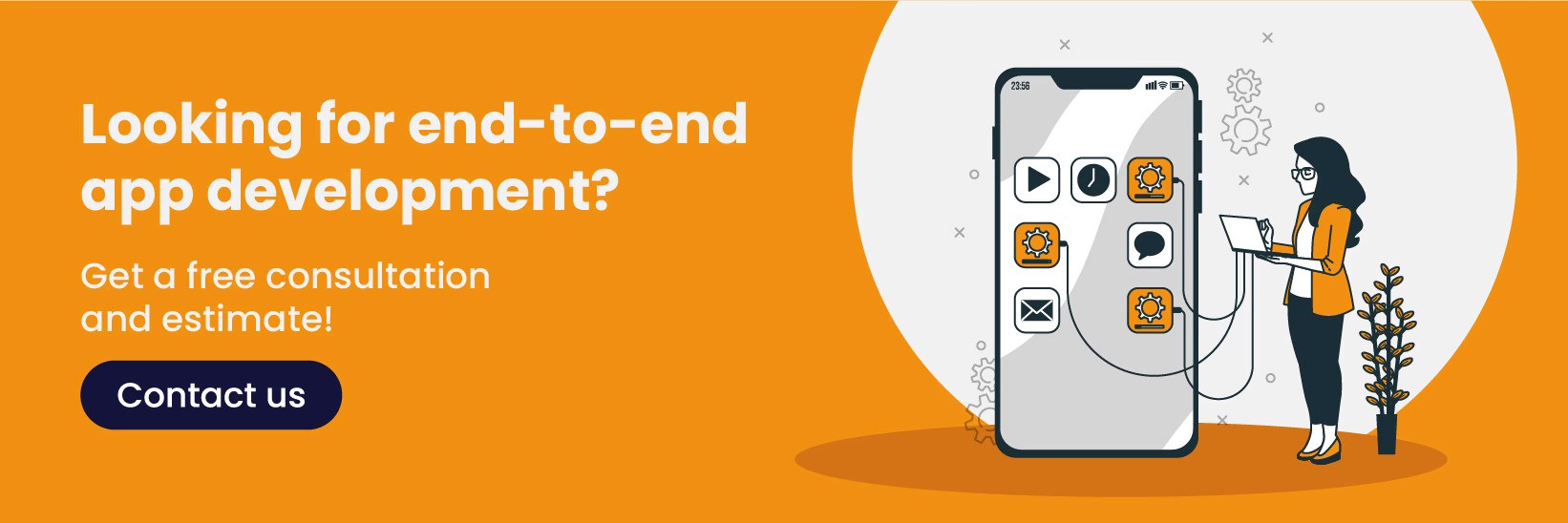
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
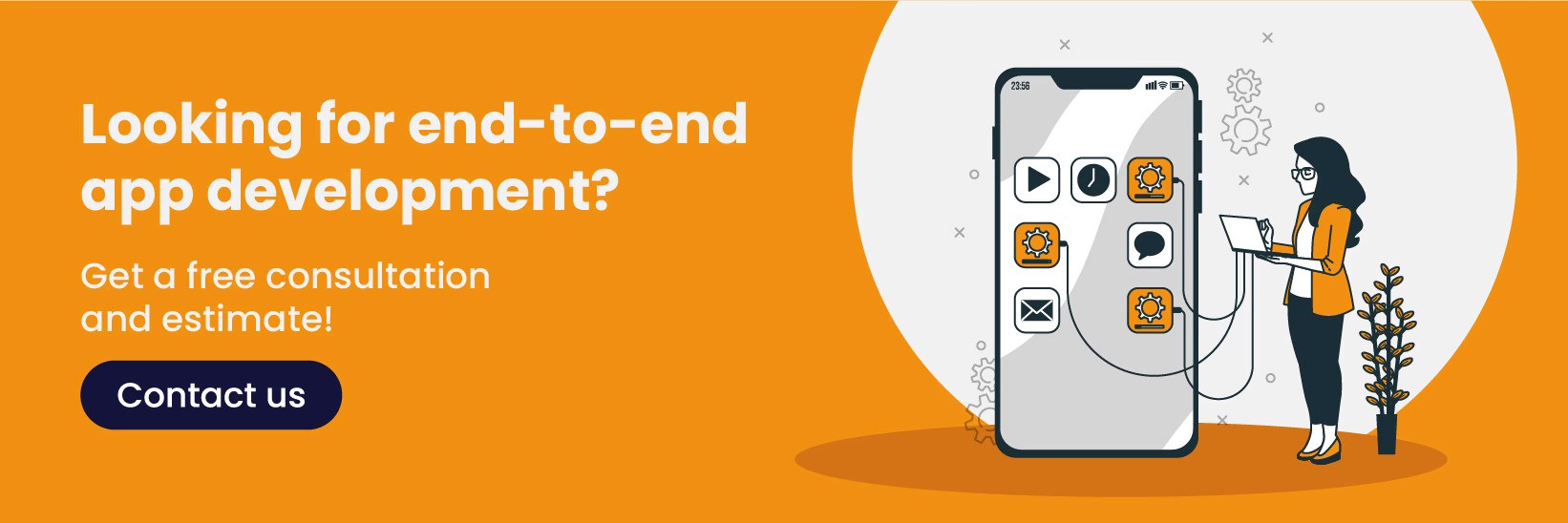
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
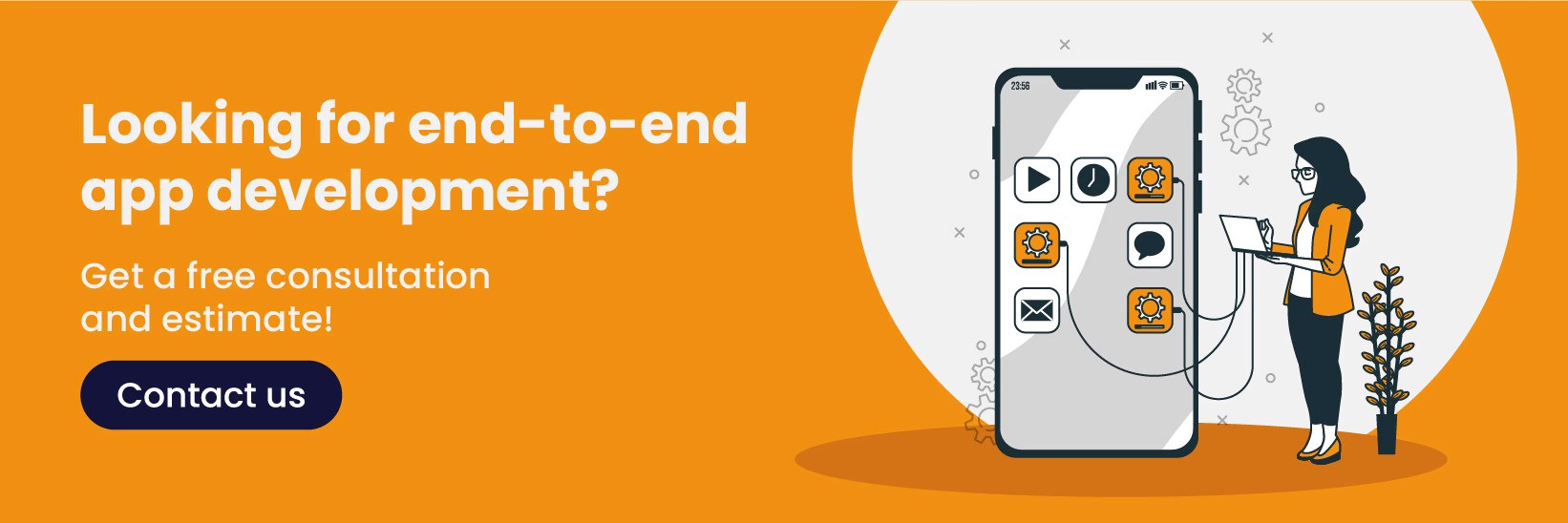
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Building a web application in the twenty-first century implicates the use of elements that make projects in the application’s front end and back end coherent. This is where React and Python come out as the choices for the full-stack development process. Based on the data of programming languages in use today 2025, more than 40% of the developers’ preference is the use of React for front-end application Similarly, there is necessary information that python as one of the most desirable languages, also offers a percentile рейтинг and simplicity.
ReactJS Development Services is a JavaScript library that is available for open source, this mean the builders are allowed to design the dynamic UIs without any cost. The localization of the virtual environment, working with component-based structures, sparing usage of virtual DOM, and effective state management makes it appropriate for the development of high-quality web applications and optimally responsive UIs.
On the other side, Python is also one of the high-level languages which people like due to convenience of writing it and its great opportunities which include tremendous number of libraries. Mostly, it is utilized in back end APIs and databases to machine learning and other Artificial Intelligence applications.
Why Use React with Python?

Custom and Real-Time Functionality
Among its features it provides for the state management and supports WebSocket for real time updated making React suitable for all kinds of cooperation utilities, live dashboards, etc and many more interactive kind of applications. This feature is even better with the latest event-driven frameworks such as the FastAPI for Python Development Services.
Enhanced API Integration
Well-known web app frameworks like Django REST Framework allow building REST APIs and consume those APIs efficiently, and React. When done effectively, this helps SPA s and PWA s to be free from latency issues with routing for proper handing of the data exchange for various routes.
Microservice Development
It has many features that point to the fact that it is a lightweight solution, which makes it easy to plug into the microservices architecture. Flask in particular or any framework based on Python is rather efficient for backend micro-services for the replacement of original monolithic way of application building.
Robust Web Security
Robust Web Security using React Frontend and Python Backend is equipped with protections from main vulnerabilities in frameworks like Django some of which include SQL injection and cross site scripting. To this, one can simply build on the already secure frontend supplied by React, making for the overall of the app’s security.
Cost-Effective Development
The second is that the use of the React library of reusable component combined with the simplicity of Python reduces the time and efforts needed to develop the application. That makes the stack perfect for startups and companies that want to launch MVPs as soon as possible.
Future-Ready Architecture
Even though the level of reusability and the level of controls provided by React are remarkable and future-ready Architecture with React and Python, React will be easily integrable with the modern Python ML capabilities and functionality.
Setting Up Your Full-Stack Environment
Designing an application for the World Wide Web today implies choosing the technology stack. .React and Python should also be listed among the top technologies because their frontend and backend aspects are very well developed. In this tutorial, you will get an understanding of how you should structure your environment for a React-Python full-stack development project.
Prerequisites
Currently before proceeding further you have to make sure that you have Node.js and npm for React.
First, by navigating the Internet, go to the official Node.js web site, where Node.js package with npm can be downloaded and installed. They are tributary of the dependencies that are also used to run a mere application in React.
Download and install Python with pip for Backend Development
Access the official site of Python to download for Python. Ensure pip is installed to handle backend libraries such as flask or Django, these are packages developed by python.
IDE Recommendations
For seamless development, use IDEs such as:
Visual Studio Code: It’s a good tool for either react or python and has many plugins.
PyCharm: Optimised for use with the Python language and comes with a set of powerful debugging facilities.
Official Setup Guides
React: Get Started with React
Python: Python Setup Guide
These tools will prime environment for creating highly innovative, highly scalable full-stack solutions with little effort.
Building the Frontend with React
React is the JavaScript library used for building user interface with huge popularity. This architecture and its state management tools make it suitable for building rich, interactive and responsive web applications. This guide will take you step-by-step on how to create your React application, creating the components, and then managing your state using React hooks.
Step 1: Initialize a React App
Begin by creating of your React project. For this purpose, you can use either create-react-app or the newer Vite.
Using create-react-app:
bash
npx create-react-app my-app
cd my-app
npm start
Using Vite:
bash
npm create vite@latest my-app --template react
cd my-app
npm install
npm run dev
Step 2: Create Components for the User Interface
The basic application of React is that its applications are developed with reusable components. For example:
jsx // src/components/Button.js import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
In your main app file, import and use the Button component:
jsx import React from 'react'; import Button from './components/Button'; function App() { return ( <div> <h1>Welcome to My React App</h1> <Button label="Click Me" onClick={() => alert('Button clicked!')} /> </div> ); } export default App;
Step 3: Manage State and Side Effects with Hooks
The react hooks help to manage the state and side effects of a code.
useState for managing state:
jsximport React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }; export default Counter;
Use Effect for handling side effects:
jsximport React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((result) => setData(result)); }, []); return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }; export default DataFetcher;
Setting Up the Backend with Python
Selecting the proper backend framework is fundamental while creating a brand-new web application in 2025. As a language, Python is particularly darling due to its simplicity and flexibility in terms of use; the primary directions would include backend, and the frameworks here are Django and Flask. The two work in their specific ways, thus providing a good opportunity for different types of projects.
Choosing Between Django and Flask
Django: For large complicated applications where common functions like security, database abstraction layer, and an administrative interface are included. This is implemented with the approach familiar to some developers as the “batteries-included” philosophy, which minimizes the requirement for extra libraries.
Flask: Customizable and more versatile specifically for small to medium size applications or where the control of every element is needed. Flask has no bloat, which makes it perfect for developers building microservices or custom APIs.
Steps to Create a Backend with Django or Flask
Create a New Project
Django:
bash
django-admin startproject myproject
cd myproject
python manage.py runserver
Flask:
bash
pip install flask
touch app.py
Add the following to app.py:
pythonfrom flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Set Up Basic API Endpoints
Django: For APIs use Django REST Framework (DRF).
bash
pip install djangorestframework
Define views and endpoints in views.py.
Flask: Use Flask’s built-in routing to define endpoints in app.py.
Connect to a Database
Django:
Use SQLite by default or if you want to use postgres it has to be configured in settings.py file.
python
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'dbname', 'USER': 'username', 'PASSWORD': 'password', 'HOST': 'localhost', 'PORT': '5432', } }
Flask: :Install and configure SQLAlchemy to allow it to talk to the database:
bash
pip install flask-sqlalchemy
Popular Python Libraries for Backend Functionality
Django REST Framework (DRF): Simplifies API development.
SQLAlchemy: A powerful ORM for Flask.
Celery: Handles asynchronous tasks.
Flask-SocketIO: Implemented WebSocket support for activity that need real time components.
When using these tools and frameworks together, you easily build up a solid backend that ties firmly with your React Frontend Development, making it easy to build scalable full-stack applications.
Integrating React with Python
The core idea of the most modern web development is based on the combination of the best technologies. Some of the most demanded ones are React and Python. React now owns the frontend world because it is a component-based framework with a virtual DOM to create reactive interfaces. Python is well-known for its simplicity and suitability for different purposes; it shines at backend logic, API, ML. Combined, they present a rich and coherent platform for highly-scalable and inventive web projects.
Why Combine React with Python?
This means that, using React and Python together offers a perfect Full Stack Development experience. React makes it easy to create and create engaging interfaces while Python takes care of backend functionalities. This combination is well suited for real-time environments, data-driven applications, and growing systems.
Step-by-Step Integration
Set Up the Python Backend
See to it that there’s Python and set up a back-end API with assistance like Flask or Django. Example Flask snippet:
python from flask import Flask, jsonify, request app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): return jsonify({"message": "Hello from Python!"}) if __name__ == '__main__': app.run(debug=True)
Develop the React Frontend
For development of the React project base, please use the create-react-app. To interface with the API you’ll need Axios for the front end to receive data from the python end. Example React code:
javascriptimport React, { useState, useEffect } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState(''); useEffect(() => { axios.get('http://127.0.0.1:5000/api/data') .then(response => setData(response.data.message)) .catch(error => console.error(error)); }, []); return <div><h1>{data}</h1></div>; }; export default App;
Address CORS Issues
They should improperly setup Flask or Django to accept cross origins while using such libraries as
flask-cors or django-cors-headers.
Benefits of Integration
Custom Functionality: Develop real- time insights such as live performance reports and related collaboration tools.
API Efficiency: Python frameworks make API development easy, and React savor them effortlessly.
Scalability: One can easily scale with React’s reusable components as well as Python’s microservices architecture.
Future-Proofing: Leverage Python AI/ML for applications with React’s UX for modern day applications.
Tools and Frameworks to Enhance Full-Stack Development
Building a next generation web app requires usage of development tools and frameworks to simplify both the frontend and backend development. React and Python have become favorites in the tech stack for the present days. It is used for dynamic user interfaces, that is why according to the 2025 StackOverflow React got higher rate among other frameworks and libraries – over 40 percent; Python is famous for easy or rather simple code and great opportunities in backend and data analysis.
React: React is a JavaScript library based which provide some of the features like component reusability, a fast DOM interface, and the capability to run on both web and mobile. It is based on a modular architecture that enhances productivity in development and brings UTI management in focus.
Python: With a having a clean syntax and the availability of large libraries, Python can support backends such as Flask and Django, which are ideal for building strong API’s and data manipulation. It is also crucial for building machine learning interfaces and for managing real-time applications.
The Synergy: React works perfectly with Python to give a perfect Full Stack Development environment. React is responsible for handling dynamic component while Python will efficiently handle server based processes. This duo offers extensible, resilient, and future-proof applications that meet the demand for enabling advanced web solutions.
Check out this tech stack and start using it in your project – it will redefine your approaches to development.
Best Practices for React + Python Full-Stack Development
To create web applications on the basis of React and Python, one has to deal with the strengths of both the technologies. React makes it possible to maintain versatile and agile interfaces, and Python is assigned to implement solid business functionalities. Here are best practices to streamline development:
Modularity: So, for better debugging and scaling, keep React and Python projects in parts.
API Communication: APIs must use HTTPS as a method of accessing web services and use tokens as the means of authentication.
Reusable Components: Refactor the code to make React components reusable/ React components and make Python code easily form modular.
Error Handling: Ensure that there is great and secure error management at both the client side and the server side.
Optimize Performance: We would recommend to use caching, pagination and Docker for deployment.
JSON Standards: To keep up with the continuous communication, JSON should remain the default data format to use.
Adopting these practices makes the development efficient and there is mechanism for future scaling which makes React and Python the ideal tech stack for modern Full Stack Development apps.
Conclusion
Using React for front-end and Python for the back end or full-stack makes the system highly manageable. React is used for creating the interactive and dynamic user interface because it has component based on Virtual DOM and with Python, you have numerous libraries and frameworks for creating back end such as Django and Flask. Alongside, they allow for integration of APIs, for enabling microservices architecture and highly, adaptive real-time applications.
This synergy helps in consolidating work flows and increasing Performance Optimization rates, and minimizing the development time, thus it is suitable for projects like data driven web apps, E-commerce platforms and AI solutions. Make the best of this future practical stack to build strong, safe, and user-driven web applications at scale.
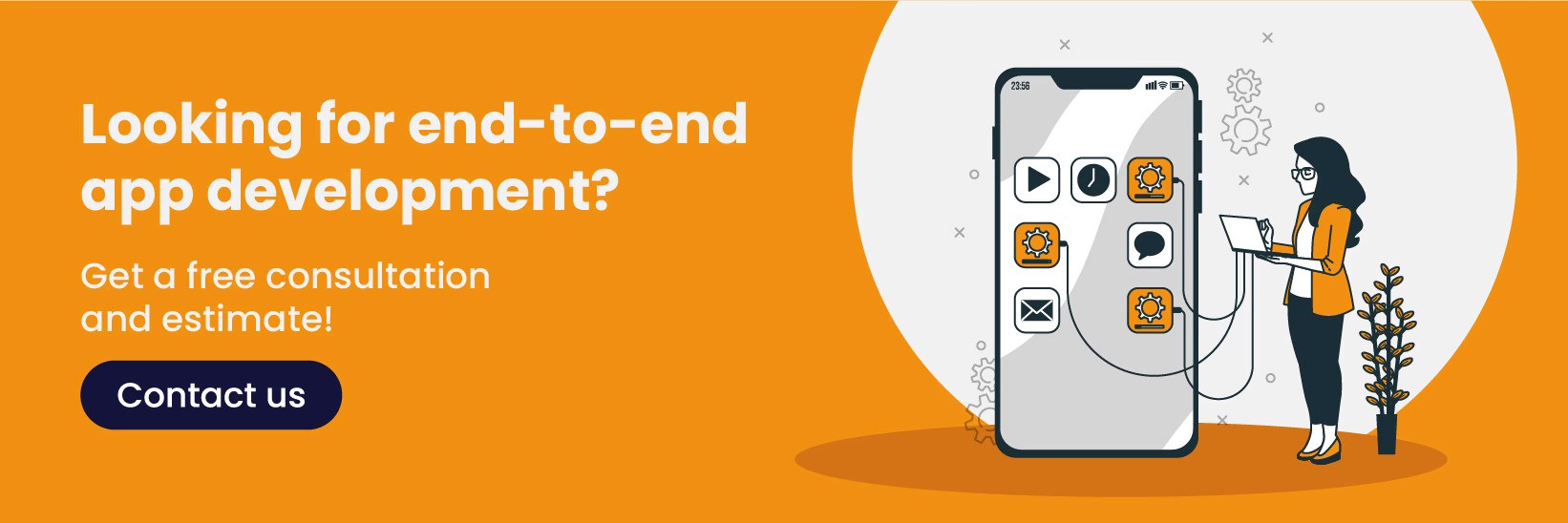
FAQs
Q: How does integrating React with Python for Web App Development enhance scalability?
A: React’s component-based architecture and Python’s support for microservices enable seamless scalability.
Q: What challenges arise in integrating React with Python?
A: Common challenges include CORS configuration and managing asynchronous data flows. Proper middleware setup and API design mitigate these issues.
Q: Can React be used with any Python framework?
A: Yes, React integrates well with frameworks like Django, Flask, and FastAPI.
Q: How do you handle data security in a React and Python app?
A: Use HTTPS, token-based authentication, and backend frameworks’ built-in security features.
Recent Posts
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India
Ai
Services
Technologies
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India
Ai
Services
Technologies
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India
Technologies
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India
Ai
Services
Technologies
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India
Ai
Services
Technologies
Transform your vision into reality with Custom Software Development
Get Started

Office Address:
743A, Gera’s Imperium Rise,Hinjewadi Phase II, Rajiv Gandhi Infotech Park, Near Wipro Circle, Pune- 411057, Maharashtra, India